in this article, i will guide you how to setup / installation of laravel reverb to make real-time chat app easily. It’s super easy to setup and make real-time chat web application with livewire.
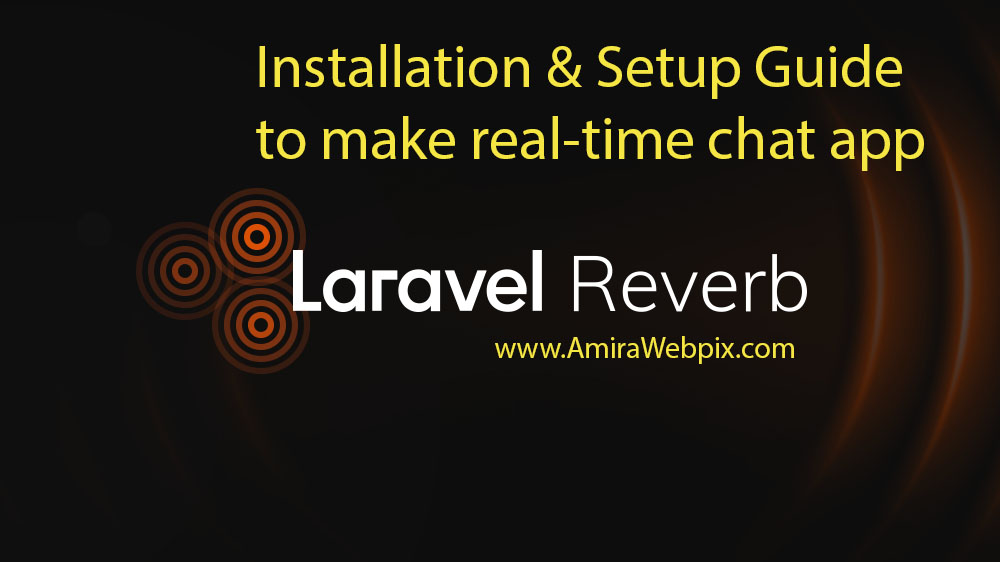
Laravel Reverb is a game-changer for building chat apps and other real-time features within your Laravel applications. This official Laravel package acts like a dedicated server for websockets, enabling instant two-way communication between your web pages and the Laravel application. With Reverb, messages can be sent and received in real-time, keeping your app feeling alive and responsive.
Reverb is only supported with Laravel v11.x. so first of all, you have to install laravel 11 with the following command.
composer create-project laravel/laravel:^11.0 my-chat-app
After a fresh installation of laravel 11, you can install starter kit of laravel breeze for basic authentication.
composer require laravel/breeze --dev
After Composer has installed the Laravel Breeze package, you should run the breeze:install Artisan command. The breeze:install command will prompt you for your preferred frontend stack and testing framework:
php artisan breeze:install

I will choose Livewire. You can choose any one of them.
Now it’s time to install Laravel reverb with the following command:-
php artisan install:broadcasting

type “yes”, after then it will ask to install and build the node dependencies for broadcasting.

This command will install all dependencies like pusher-js, laravel-echo that is required for reverb. The Broacasting config file and channels route file will be also published.
Now open .env file and set QUEUE_CONNECTION value to “sync” like this:-

It is required becasue if you leave it default as “database”. you will need to run extra command “queue:work”. if you set this as “sync” then no queue command needed.
The Reverb server can be started using the reverb:start Artisan command:
php artisan reverb:start
By default, the Reverb server will be started at 0.0.0.0:8080, making it accessible from all network interfaces. If you need to specify a custom host or port, you may do so via the –host and –port options when starting the server:
php artisan reverb:start --host=127.0.0.1 --port=9000
I would suggest to run reverb start command with debug option
php artisan reverb:start --debug
this will output debug information related to broadcasting events in terminal.
Since Reverb is a long-running process, changes to your code will not be reflected without restarting the server via the reverb:restart Artisan command.
php artisan reverb:restart
Now your reverb server is ready to send and receive real-time messages by using laravel events.
it’s time to coding. You have to create event file to send messages with the following command:-
php artisan make:event SendMessage
It will create SendMessage.php file in the App/Events Folder. Don’t forget to add ShouldBroadcast class, a parameter in construct method and rename your channel name as follow:-

We will use Public Channel at this time for testing purpose. you can replace “public-channel” with any channel name you like.
Now we will create livewire component to send and receive message. just run this command with your component name you like.
php artisan make:livewire ChatBox
we will also create seprate app.blade.php file of livewire layout.
php artisan livewire:layout
Open app.blade.php layout file from views/components folder and replace with the code
<!DOCTYPE html>
<html lang="{{ str_replace('_', '-', app()->getLocale()) }}">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>{{ $title ?? 'Page Title' }}</title>
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@4.6.2/dist/css/bootstrap.min.css">
@vite(['resources/css/app.css', 'resources/js/app.js'])
</head>
<body>
<div class="container">
{{ $slot }}
</div>
</body>
</html>
I just added bootstrap and @vite js files to include laravel-echo in the app layout. don’t forget to run node build command.
npm run build
Now open chat-box.blade.php file of livewire and add the following code.
<div>
<div class="row">
<div class="col-12 col-md-6 mx-auto mt-5 p-5 border">
<form wire:submit="submit">
<div class="form-group">
<input type="text" class="form-control form-control-lg" wire:model="message" placeholder="your message here..">
</div>
<button type="submit" class="btn btn-dark px-5 btn-lg">SEND</button>
</form>
</div>
</div>
</div>
in the last, open ChatBox.php file and replace with the following:-
<?php
namespace App\Livewire;
use Livewire\Component;
use App\Events\SendMessage;
use Livewire\Attributes\On;
class ChatBox extends Component
{
public $message;
public function submit(){
SendMessage::dispatch($this->message);
$this->message = '';
}
#[On('echo:public-channel,SendMessage')]
public function onMessageReceived($event){
dd($event);
// $this->js("alert('message received!')");
}
public function render()
{
return view('livewire.chat-box');
}
}
Now it’s time to test it in the browser and the result will awesome.

if you have any issue regarding this tutorial. you can comment me. This one is a basic chat app on public channel. if you need more advance real-time chat with auth login then please comment.
I'm Murad Saifi, an experienced freelance website designer and developer with over 5 years of industry expertise. Specializing in PHP and the Laravel Framework, I've successfully delivered diverse projects, including e-commerce websites, CRM, CMS platforms, matrimony website and web portals. With skills in crafting secure back-end panels, integrating APIs, and payment gateways, I ensure seamless functionality.
During my free time, I enjoy writing blogs on various topics like web technology, internet trends, android, website security, AI, and ChatGPT. With a deep passion for the industry and a commitment to staying up-to-date, I share valuable insights and expertise on my blog. Join me as we explore the ever-evolving world of web design, development, and the exciting realm of technology.